Zhiguang Huo (Caleb)
Monday Oct 17th, 2022
Recommended approach in this class
# Header 1
## Header 2
### Header 3
#### Header 4
##### Header 5
###### Header 6
Plain text
End a line with two spaces to start a new paragraph.
*italics* and _italics_
**bold** and __bold__
<span style="color:red">color</span>
superscript^2^
~~strikethrough~~
[link](www.rstudio.com)
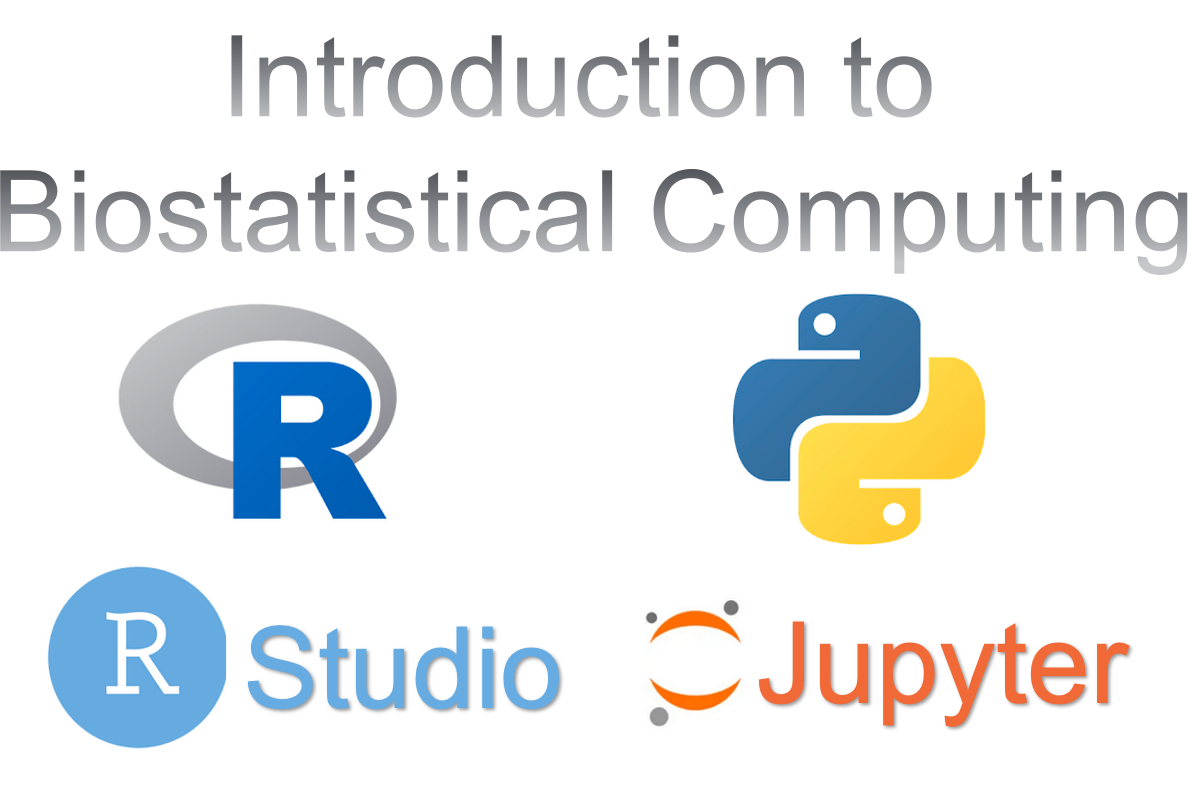{width=50%}
$A = \pi \times r^{2}$
***
* unordered list
* item 2
+ sub-item 1
+ sub-item 2
1. ordered list
2. item 2
+ sub-item 1
+ sub-item 2
print("hello")
print("hello")
python hello.py
## <class 'int'>
## <class 'float'>
## <class 'str'>
## <class 'bool'>
type(False)
## 1
## '1.1'
## 3.15
## 1
## False
## 'False'
## 3
## 'Alice'
## 'Carl'
## ['Alex', 'Beth', 'Carl']
## 3
## 'Alice'
## 'Carl'
names[0] = "Alex"
names
## (3, 1)
## [3, 1]
## [1, 3]
## 3
## 1
## 2
## [2, 3, 4]
## 3
## {'Alice': 2341, 'Beth': 4971, 'Carl': 9401}
## 2341
## {'Name': 'Smith', 'Age': 44}
## {'Name': 'Smith', 'Age': 44}
## 8
## 16
## 1
## 'HelloWorld'
## False
## True
## 9
## 'g'
## 's'
## 'gree'
## 't'
## HelloWorld
## HelloWorld
## 'reetings'
## 'gre'
## 'greeting'
## 'getn'
## 'sgniteer'
## 'sgniteerg'
## 'HiHiHi'
## 'Hi Lucas, greetings'
## Hi Lucas
## greetings
## 11
"""
XXX
XXXX
"""
## '\nXXX\nXXXX\n'
##
## XXX
## XXXX
## Alex is 27 years old
## Alex is 27 years old
## Alex is 27 years old
We will focus on the f-string in this class
f'{}'
## There are total of 40 apples
## There are 4 bags, and 10 apples in each bag.
## So there are a total of 40 apples
## 12.30
## 12.30000
## 12.3
## 12.3
## 00012.3
for x in range(1, 11):
print(f'{x:02} {x*x:3} {x*x*x:4}')
f'{value:{width}.{precision}}'
## ' 5.500'
## 3.141592653589793
## ' 3.141593'
f'{pi*100000:,.2f}'
username = input("What is your name?")
print("Hello " + username)
print(f"Hello {username}")
num1 = input("First number:")
num2 = input("Second number:")
res = int(num1) + int(num2)
print(res)
print(f"{num1} plus {num2} is {res}")
## 0
## 2
## 3
## 3
## 11
## -1
title.index("love")
title.index("XX")
## True
## True
## False
## True
## True
## 2
## '1+2+3+4+5'
## '12345'
## '/usr/bin/env'
sep = "+"
print("C:" + "\\".join(dirs)) ## single \ has special meaning: treating special symbol as regular symbol
## C:\usr\bin\env
## ['1', '2', '3', '4', '5']
## ['1+2+', '+4+5']
## ['Using', 'the', 'default', 'value']
## 'i like introduction to biostatistical computing!'
## 'I LIKE INTRODUCTION TO BIOSTATISTICAL COMPUTING!'
## 'I Like Introduction To Biostatistical Computing!'
sentence.islower()
sentence.isupper()
sentence.istitle()
## 'internal whitespace is kept'
## 'SPAM * for * everyone'
## 'a\nb\n\n\nc'
## 'That is a cat!'
## 'Theez eez a cat!'
name = input("What is your name? ")
if name.endswith("Smith"):
print("Hello, Mr. Smith")
name = input("What is your name? ")
if name.endswith("Smith"):
print("Hello, Mr. Smith")
print("Have a good night")
num = input("Enter a number: ")
if num > 0:
print("The number is positive")
else:
print("The number is non-positive")
num = input("Enter a number: ")
if num > 0:
print("The number is positive")
elif num < 0:
print("The number is negative")
else:
print("The number is zero")
name = input("What is your name? ")
if name.endswith("Smith"):
if name.startswith("Mr."):
print("Hello, Mr. Smith")
elif name.startswith("Mrs."):
print("Hello, Mrs. Smith")
else:
print("Hello, Smith")
else:
print("Hello, Stranger")
number = input("Please enter a number: ")
if int(number) % 2 == 0:
print("even")
else:
print("odd")
number = input("Please enter a number: ")
print("even") if int(number) % 2 == 0 else print("odd")
## False
## False
## True
## True
## False
## True
## False
## True
## True
## True
status = 400
match status:
case 400:
print("Bad request")
case 404:
print("Not found")
case 418:
print("I'm a teapot")
case _:
print("Something's wrong with the internet")
## cat
## dog
## gator
## cat has 3 letters in it.
## dog has 3 letters in it.
## gator has 5 letters in it.
## 0
## 1
## 2
## [0, 1, 2]
## [3, 4, 5, 6]
## [3, 5, 7]
## [7, 5, 3]
## 0 cat
## 1 dog
## 2 gator
for num in range(1, 10):
if num % 5 == 0:
print(f"{num} can be divided by 5")
break
print(f"{num} cannot be divided by 5")
## 1 cannot be divided by 5
## 2 cannot be divided by 5
## 3 cannot be divided by 5
## 4 cannot be divided by 5
## 5 can be divided by 5
## 1 cannot be divided by 5
## 2 cannot be divided by 5
## 3 cannot be divided by 5
## 4 cannot be divided by 5
## 6 cannot be divided by 5
## 7 cannot be divided by 5
## 8 cannot be divided by 5
## 9 cannot be divided by 5
num = 1
while num<10:
if num % 5 == 0:
print(f"{num} can be divided by 5")
break
print(f"{num} cannot be divided by 5")
num+=1
## 1 cannot be divided by 5
## 2 cannot be divided by 5
## 3 cannot be divided by 5
## 4 cannot be divided by 5
## 5 can be divided by 5
## 1 cannot be divided by 5
## 2 cannot be divided by 5
## 3 cannot be divided by 5
## 4 cannot be divided by 5
## 6 cannot be divided by 5
## 7 cannot be divided by 5
## 8 cannot be divided by 5
## 9 cannot be divided by 5
https://caleb-huo.github.io/teaching/data/misc/my_file.txt
## Hello, my name is Caleb. Hello World!
## I like computing
## Hello, my name is Caleb. Hello World!
## I like computing
myfile = "my_file.txt"
with open(myfile) as file:
lines = file.readlines()
for aline in lines:
print(aline.strip())
## Hello, my name is Caleb. Hello World!
## I like computing
## 32
## 33
with open("a_file.txt") as file:
file.read()
fruit_list = ["Apple", "Banana", "Pear"]
fruit_list[3]
text = "abc"
print(text + 5)
raise TypeError("This is an error that I made up!")
try:
file = open("a_file.txt")
print(1 + "2")
except FileNotFoundError:
print("Catch FileNotFoundError")
except TypeError as error_message:
print(f"Here is the error: {error_message}.")
else:
content = file.read()
print(content)
finally: ## will happen no matter what happens
file.close()
print("File was closed.")
## Catch FileNotFoundError
## File was closed.
## 2022
## 10
## 17
## 9940