Zhiguang Huo (Caleb)
Tuesday Oct 10th, 2023
Recommended approach in this class
# Header 1
## Header 2
### Header 3
#### Header 4
##### Header 5
###### Header 6
Plain text
End a line with two spaces to start a new paragraph.
*italics* and _italics_
**bold** and __bold__
<span style="color:red">color</span>
superscript^2^
~~strikethrough~~
[link](www.rstudio.com)
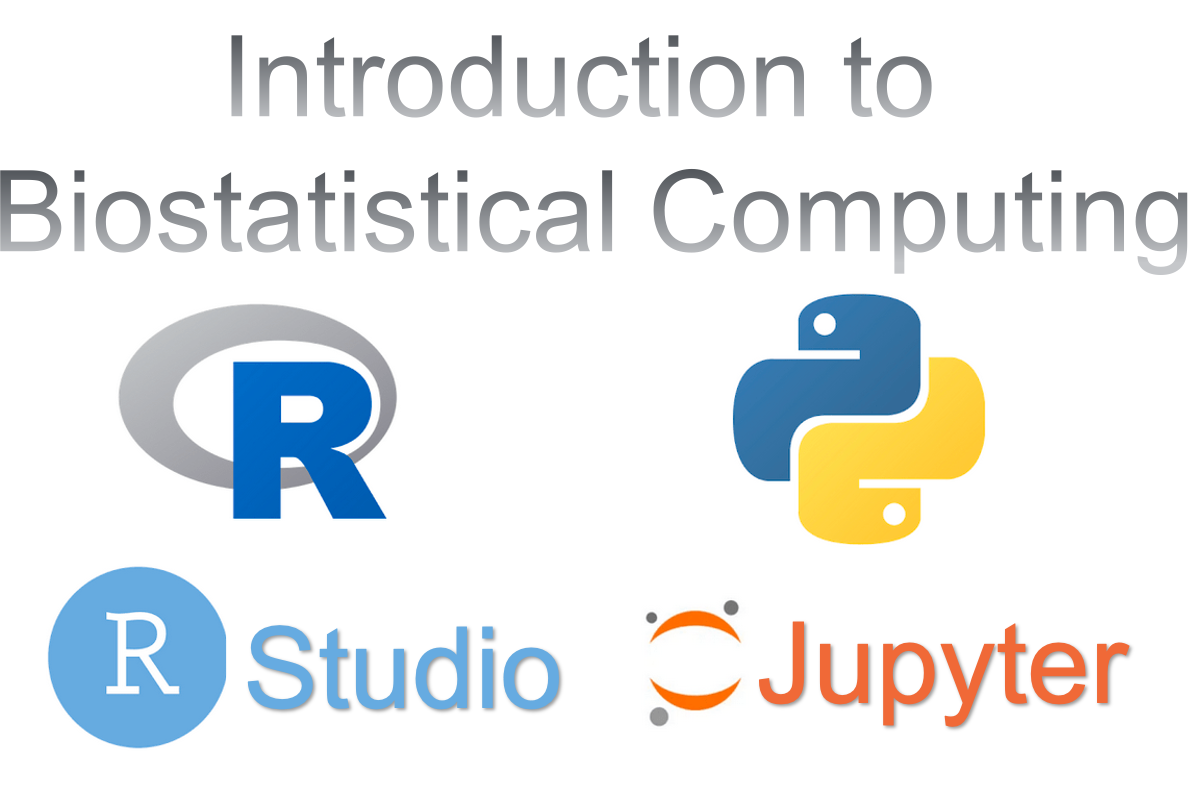{width=50%}
$A = \pi \times r^{2}$
***
* unordered list
* item 2
+ sub-item 1
+ sub-item 2
1. ordered list
2. item 2
+ sub-item 1
+ sub-item 2
print("hello")
print("hello")
python hello.py
## <class 'int'>
## <class 'float'>
## <class 'str'>
## <class 'bool'>
type(False)
## 1
## '1.1'
## 3.15
## 1
## False
## 'False'
## 3
## 'Alice'
## 'Carl'
## ['Alex', 'Beth', 'Carl']
## 3
## 'Alice'
## 'Carl'
names[0] = "Alex"
names
## (3, 1)
## [3, 1]
## [1, 3]
## 3
## 1
## 2
## [2, 3, 4]
## 3
## {'Alice': 2341, 'Beth': 4971, 'Carl': 9401}
## 2341
## {'Name': 'Smith', 'Age': 44}
## {'Name': 'Smith', 'Age': 44}
## 8
## 16
## 1
## 'HelloWorld'
## False
## True
## 9
## 'g'
## 's'
## 'gree'
## 't'
## HelloWorld
## HelloWorld
## 'reetings'
## 'gre'
## 'greeting'
## 'getn'
## 'sgniteer'
## 'sgniteerg'
## 'HiHiHi'
## 'Hi Lucas, greetings'
## Hi Lucas
## greetings
## 11
"""
XXX
XXXX
"""
## '\nXXX\nXXXX\n'
##
## XXX
## XXXX
## Alex is 27 years old
## Alex is 27 years old
## Alex is 27 years old
We will focus on the f-string in this class
f'{}'
## There are total of 40 apples
print(f'There are {bags} bags, and {apples_in_bag} apples in each bag.\nSo there are a total of {bags * apples_in_bag} apples')
## There are 4 bags, and 10 apples in each bag.
## So there are a total of 40 apples
## 12.30
## 12.30000
## 12.3
## 12.3
## 00012.3
for x in range(1, 11):
print(f'{x:02} {x*x:3} {x*x*x:4}')
f'{value:{width}.{precision}}'
## ' 5.500'
## 3.141592653589793
## ' 3.141593'
f'{pi*100000:,.2f}'
username = input("What is your name?")
print("Hello " + username)
print(f"Hello {username}")
num1 = input("First number:")
num2 = input("Second number:")
res = int(num1) + int(num2)
print(res)
print(f"{num1} plus {num2} is {res}")